jvalero
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-22-2012
11:32 AM
roUrlTransfer - Problems with multiple URLs
I'm having a problem which I'd like to see if the community can help me solve. It has to do with a very important part of my company's Roku channels, mainly stat tracking or ad playback. Let me quickly walk you through the general setup and how things are supposed to work.
Each time we play a content video, the channel fires off to our ad server to see if there is a preroll ad to playback before the content video begins. So the channel parses through the XML response from our ad server and pulls out impression URLs as well as what we refer to as quartile stat urls. Impression URLs are fired at the beginning of playback, quartile stats are fired at the start, 25%, 50%, 75% and complete markers. There can be several URLs for each marker or just one. So what happens is the XML parser pushes the URLs into an array for each marker. That part is working properly and when I watch ad playback through the debug console I get 200 responses for each URL pinged.
However, for some reason the stats don't equal out. Now in a live environment this wouldn't be so important, as someone might not watch the entire ad. They may exit the app or something else might happen to interfere with playback. However, we're testing this on a staging app that only we have access to and each ad is played back for its entirety each time playback occurs while we're testing. Even worse is that what our start marker stats seem to be lower than our completes. So something is happening where not all URL pings are getting through even though the response shows successful in the debugger.
So now for the specifics of what is happening in the code. During playback we check for each marker, and once it is hit all the associated URL pings are fired off. This only happens the first time as we have a boolean setup to only enter the loop if not fired before. Previously we ran into the issue where our URL transfers were walking over each other. We fixed this by creating a function to track and handle unique instances of roUrlTransfer objects for each URL ping that occurs. Here is that function:
And here is the other side of it where we close things out in our event loop:
Can anyone see any reason from the code above, or have any idea what might be blocking the pings to the URLs, if in fact I'm seeing all of the the correct things happening in the debugger, and the responses are coming back as they should? I appreciate the help in advance.
Each time we play a content video, the channel fires off to our ad server to see if there is a preroll ad to playback before the content video begins. So the channel parses through the XML response from our ad server and pulls out impression URLs as well as what we refer to as quartile stat urls. Impression URLs are fired at the beginning of playback, quartile stats are fired at the start, 25%, 50%, 75% and complete markers. There can be several URLs for each marker or just one. So what happens is the XML parser pushes the URLs into an array for each marker. That part is working properly and when I watch ad playback through the debug console I get 200 responses for each URL pinged.
However, for some reason the stats don't equal out. Now in a live environment this wouldn't be so important, as someone might not watch the entire ad. They may exit the app or something else might happen to interfere with playback. However, we're testing this on a staging app that only we have access to and each ad is played back for its entirety each time playback occurs while we're testing. Even worse is that what our start marker stats seem to be lower than our completes. So something is happening where not all URL pings are getting through even though the response shows successful in the debugger.
So now for the specifics of what is happening in the code. During playback we check for each marker, and once it is hit all the associated URL pings are fired off. This only happens the first time as we have a boolean setup to only enter the loop if not fired before. Previously we ran into the issue where our URL transfers were walking over each other. We fixed this by creating a function to track and handle unique instances of roUrlTransfer objects for each URL ping that occurs. Here is that function:
Sub PingURL(urlToCall as String, activeURLArray as Object, messagePort as Object)
'// Create a urlTransfer, store in the array of atcive transfers to keep a reference to it, and Activate it.
transfer = CreateObject("roUrlTransfer")
transfer.SetPort(messagePort)
transfer.SetUrl(urlToCall)
activeURLArray.push(transfer)
PrettyPrint("Starting PingURL() Call, uniqueid="+transfer.GetIdentity().ToStr()+": " + urlToCall) '// Custom function for creating a custom print block
transfer.AsyncGetToString()
End Sub
And here is the other side of it where we close things out in our event loop:
While True
'// Check the urlTransfer port for any updates on UrlTransfers
msg = wait(100, urlTransferPort)
'// URL Transfer Events
If Type(msg) = "roUrlEvent"
Print "--[roUrlEvent. uniqueid="+msg.GetSourceIdentity().ToStr()+" type="+ msg.GetInt().ToStr() + " responsecode="+msg.GetResponseCode().ToStr()+" statusText="+msg.GetFailureReason()+" targetIP="+msg.GetTargetIpAddress()+"]"
'//Loop over the array of all active roURLTransfers, looking for the mathc that this event was generated for.
For i=0 to m.activeUrlTransfers.Count()-1 step +1
If m.activeUrlTransfers[i].GetIdentity() = msg.GetSourceIdentity()
'// Check if the transfer has finished - it will have a value of 1 if it is finished
If msg.GetInt() = 1 Then
'//Remove the transfer from the array of active URLs.
'//Remove this entry from the array.
m.activeUrlTransfers.Delete(i)
Print "active URL complete, removing, active URLs now left: "+m.activeUrlTransfers.Count().ToStr() + ""
m.responseCount = m.responseCount + 1
'//Break out of loop - no more checking required
Exit For
End If
End If
End For
If m.responseCount = m.totalImpressionUrls
PrettyPrint( "" + m.responseCount.toStr() + " out of " + m.totalImpressionUrls.toStr() + " ping responses received successfully. Ad playback complete. " )
Exit While
End If
Else
End If
End While
Can anyone see any reason from the code above, or have any idea what might be blocking the pings to the URLs, if in fact I'm seeing all of the the correct things happening in the debugger, and the responses are coming back as they should? I appreciate the help in advance.
6 REPLIES 6
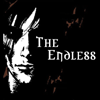
TheEndless
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-22-2012
05:44 PM
Re: roUrlTransfer - Problems with multiple URLs
Just to clarify... you're seeing 200 results for all URLs in the debugger, indicating that the requests were successful, but the tracking system isn't matching up? If that's the case, are you sure the issue isn't on the server side? Is it possible it returns a 200 result, even if the request itself fails?
My Channels: http://roku.permanence.com - Twitter: @TheEndlessDev
Instant Watch Browser (NetflixIWB), Aquarium Screensaver (AQUARIUM), Clever Clocks Screensaver (CLEVERCLOCKS), iTunes Podcasts (ITPC), My Channels (MYCHANNELS)
Instant Watch Browser (NetflixIWB), Aquarium Screensaver (AQUARIUM), Clever Clocks Screensaver (CLEVERCLOCKS), iTunes Podcasts (ITPC), My Channels (MYCHANNELS)
jvalero
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-23-2012
08:53 AM
Re: roUrlTransfer - Problems with multiple URLs
First off, I appreciate the response. I believe you've helped me in the past at least once or twice.
Basically it's firing off to impression pixels, so there isn't any processing being done other than a pixel being hit. Each marker fires off to a unique URL, with a unique pixel. These URLs from what I've seen are accessible at all times. We've also looked at some tcpdump logs from our box and saw the proper response going out and coming back in with each sample we viewed. The only thing we haven't done is try and capture all of the logs for the duration of our test period and compare it against the results to see if for some reason some don't make it through. This is because of the seemingly short lifecycle of rotating logs available from Roku. e.g. ( http://IP.ADD.RE.SS/pkgs/log0, log1, log3, log4 ). Any suggestions for how to make that experience a little more user-friendly would also be welcomed.
Basically it's firing off to impression pixels, so there isn't any processing being done other than a pixel being hit. Each marker fires off to a unique URL, with a unique pixel. These URLs from what I've seen are accessible at all times. We've also looked at some tcpdump logs from our box and saw the proper response going out and coming back in with each sample we viewed. The only thing we haven't done is try and capture all of the logs for the duration of our test period and compare it against the results to see if for some reason some don't make it through. This is because of the seemingly short lifecycle of rotating logs available from Roku. e.g. ( http://IP.ADD.RE.SS/pkgs/log0, log1, log3, log4 ). Any suggestions for how to make that experience a little more user-friendly would also be welcomed.
renojim
Community Streaming Expert
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-23-2012
01:12 PM
Re: roUrlTransfer - Problems with multiple URLs
You might want to connect your Roku through something that allows you to capture all the traffic. I've used Virtual Router and Wireshark running on my laptop to accomplish this and I'm sure there's other ways to do it.
-JT
-JT
Roku Community Streaming Expert
Help others find this answer and click "Accept as Solution."
If you appreciate my answer, maybe give me a Kudo.
I am not a Roku employee.
Help others find this answer and click "Accept as Solution."
If you appreciate my answer, maybe give me a Kudo.
I am not a Roku employee.
jvalero
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-23-2012
01:49 PM
Re: roUrlTransfer - Problems with multiple URLs
We've done this in the past using Charles, however, it isn't the easiest approach going that route as you have to have a computer with two network cards to make it work. I may have a look at Virtual Router soon, so thanks for the link. At the moment I'm using the tcpdump logs that can be enabled on the Roku box. I'm in the process of writing a shell script to fetch the files once they've completed a cycle, that I can easily deploy while testing.
From what I've noticed a it seems to cycle to the next log file once it goes over 1000000 bytes.
From what I've noticed a it seems to cycle to the next log file once it goes over 1000000 bytes.
jvalero
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-24-2012
12:27 PM
Re: roUrlTransfer - Problems with multiple URLs
Just a quick update...
The shell script route was unsuccessful. I was able to quickly grab the logs, but trying to capture them exactly at the right time and having to parse through 100s of different files just for a few minutes worth of data required too much overhead. I opted to instead setup a WiFi Hotspot on my MacBook Pro and the connected my Roku box to that network. I could then listen in on the traffic live using Wireshark.
We just ran a test during the 11AM PST hour, and I'm just waiting for the numbers to come in from our stat server. I'll provide an update once I've had a chance to analyze the data.
The shell script route was unsuccessful. I was able to quickly grab the logs, but trying to capture them exactly at the right time and having to parse through 100s of different files just for a few minutes worth of data required too much overhead. I opted to instead setup a WiFi Hotspot on my MacBook Pro and the connected my Roku box to that network. I could then listen in on the traffic live using Wireshark.
We just ran a test during the 11AM PST hour, and I'm just waiting for the numbers to come in from our stat server. I'll provide an update once I've had a chance to analyze the data.
jvalero
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-29-2012
12:24 PM
Re: roUrlTransfer - Problems with multiple URLs
Just a quick update. We're continuing to test this via Wireshark. What we've noticed is that the requests do seem to be made but we don't get a response back on some random occasions. We can see the request in Wireshark, but literally nothing comes back in these instances. No response at all. At the moment we're running Wireshark on both ends to try and see if there is an issue we can catch. There doesn't seem to be any problems with our servers so far but we're continuing to test. If anything I'm saying seems to ring any bells with anyone please post. Also, it would be nice if someone from Roku could way in on the situation. Have you run into similar issues with other publishers and apps? Any advice?