rsromeo
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-28-2010
04:58 PM
SEARCH FUNCTIONALITY EXAMPLE?
Hi -
I'm still working on learning BrightScript but not certain how to implement Search functionality into my app. Does anyone have an example of how to do this? A search example wasn't included with the SDK. I want to use roSearchScreen to allow user to performa simple search on my channel for a specific program. Thanks.
I'm still working on learning BrightScript but not certain how to implement Search functionality into my app. Does anyone have an example of how to do this? A search example wasn't included with the SDK. I want to use roSearchScreen to allow user to performa simple search on my channel for a specific program. Thanks.
7 REPLIES 7
Anonymous
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-28-2010
05:26 PM
Re: SEARCH FUNCTIONALITY EXAMPLE?
Exactly how you'd implement it depends on the search API provided by host/CMS/video platform/whatever.
rsromeo
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-28-2010
05:43 PM
Re: SEARCH FUNCTIONALITY EXAMPLE?
is there an example anywhere of how a search could be implemented using any search API? I'm just looking for ideas on how it could be done since I'm still learning. I copied the roSearchScreen and roKeyboardScreen code but don't really know what to do with them now. Appreciate any help.
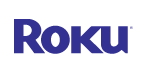
RokuKevin
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-29-2010
06:35 PM
Re: SEARCH FUNCTIONALITY EXAMPLE?
You do not need to use the roKeyboardScreen component. The roSearchScreen component includes a keyboard for text entry. The Component Reference document includes the BrightScript sample code for using the roSearchScreen and roSearchHistory components in your channel.
Most of the search functionality will be implemented on your server. In the code below, you could replace the function GenerateSearchSuggestions() with a call that retrieves search results and still returns the string array of results. The showSearchScreen() function returns the final selected search result.
Typically, you would take the final selected search result returned by searchScreen() and send that to your server to get a list of search results that you could use to fill a poster screen with content.
--Kevin
Most of the search functionality will be implemented on your server. In the code below, you could replace the function GenerateSearchSuggestions() with a call that retrieves search results and still returns the string array of results. The showSearchScreen() function returns the final selected search result.
Typically, you would take the final selected search result returned by searchScreen() and send that to your server to get a list of search results that you could use to fill a poster screen with content.
--Kevin
'*************************************************************
'** GenerateSearchSuggestions()
'*************************************************************
Function GenerateSearchSuggestions(partSearchText As String) As Object
suggestions = CreateObject("roArray", 1, true)
length = len(partSearchText)
if length > 6
suggestions.Push("ghost in the shell")
suggestions.Push("parasite dolls")
suggestions.Push("final fantasy")
suggestions.Push("ninja scroll")
suggestions.Push("space ghost")
suggestions.Push("hellboy")
else if length > 5
suggestions.Push("parasite dolls")
suggestions.Push("final fantasy")
suggestions.Push("ninja scroll")
suggestions.Push("space ghost")
suggestions.Push("hellboy")
else if length > 4
suggestions.Push("final fantasy")
suggestions.Push("ninja scroll")
suggestions.Push("space ghost")
suggestions.Push("hellboy")
else if length > 3
suggestions.Push("ninja scroll")
suggestions.Push("space ghost")
suggestions.Push("hellboy")
else if length > 2
suggestions.Push("space ghost")
suggestions.Push("hellboy")
else if length > 1
suggestions.Push("hellboy")
else if length > 0
suggestions.Push("transformers")
endif
return suggestions
End Function
'*************************************************************
'** showSearchScreen()
'*************************************************************
Function showSearchScreen() As Dynamic
screen = CreateObject("roSearchScreen")
history = CreateObject("roSearchHistory")
inHistoryMode = true
port = CreateObject("roMessagePort")
screen.SetMessagePort(port)
screen.SetBreadcrumbText("Search", "With History")
screen.SetSearchTermHeaderText("Recent Searches")
screen.SetSearchButtonText("Search")
screen.SetClearButtonText("Clear history")
screen.SetClearButtonEnabled(true) 'enable for search history
screen.SetSearchTerms(history.GetAsArray())
screen.SetEmptySearchTermsText("No searches available")
screen.Show()
while true
waitformsg:
msg = wait(0, screen.GetMessagePort())
print "message received"
if type(msg) <> "roSearchScreenEvent" then
print "Unexpected message class: "; type(msg)
goto waitformsg
endif
if msg.isScreenClosed() return invalid
if msg.isPartialResult()
partialResult = msg.GetMessage()
print "partial result "; partialResult
if not isnullorempty(partialResult)
searchSuggestions = GenerateSearchSuggestions(partialResult)
printList(searchSuggestions)
if searchSuggestions <> invalid
'do SetSearchTerms() before SetEmptySearchTermsText() so that the new empty
'search terms text doesn't flash on screen
screen.SetSearchTerms(searchSuggestions)
if inHistoryMode
'do only on 1st mode switch
screen.SetSearchTermHeaderText("Search Suggestions")
screen.SetEmptySearchTermsText("No suggestions available")
screen.SetClearButtonEnabled(false) 'user cannot clear search suggestions
inHistoryMode = false
endif
endif
endif
else if msg.isFullResult()
fullSearchResult = msg.GetMessage()
print "full result: "; fullSearchResult
if not isnullorempty(fullSearchResult)
history.Push(fullSearchResult)
endif
return fullSearchResult
else if msg.isCleared()
print "history cleared"
history.Clear()
endif
end while
End Function
arifimam
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
07-28-2011
11:22 AM
Re: SEARCH FUNCTIONALITY EXAMPLE?
where do I need to enter this code? I am suing videopayer example and want to add search on poster screen, please help.
lock_4815162342
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
01-31-2014
02:30 PM
Re: SEARCH FUNCTIONALITY EXAMPLE?
I am also trying to learn how to implement the search into the videoPlayer example. Thank you for any help you can give me.
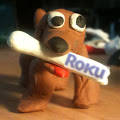
RokuJoel
Binge Watcher
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
01-31-2014
04:40 PM
Re: SEARCH FUNCTIONALITY EXAMPLE?
Since the videoplayer example is pretty much completely XML data driven, you would need to add or hijack an XML tag (and a line to parse it) to define a search item, probably in categories.xml - for example <category title="Search"
Then you would modify the brightscript code to trigger a different behavior from the norm when that item is selected, for example to show a search screen and of course you will need to create a search function that will run the search.
For example something like the modified ShowHomeScreen function below (which looks for the word "Search" in the title of the currently selected item and if it finds it, should launch a search screen function):
Then you would modify the brightscript code to trigger a different behavior from the norm when that item is selected, for example to show a search screen and of course you will need to create a search function that will run the search.
For example something like the modified ShowHomeScreen function below (which looks for the word "Search" in the title of the currently selected item and if it finds it, should launch a search screen function):
Function showHomeScreen(screen) As Integer
if validateParam(screen, "roPosterScreen", "showHomeScreen") = false return -1
initCategoryList()
screen.SetContentList(m.Categories.Kids)
screen.SetFocusedListItem(3)
screen.Show()
while true
msg = wait(0, screen.GetMessagePort())
if type(msg) = "roPosterScreenEvent" then
print "showHomeScreen | msg = "; msg.GetMessage() " | index = "; msg.GetIndex()
if msg.isListFocused() then
print "list focused | index = "; msg.GetIndex(); " | category = "; m.curCategory
else if msg.isListItemSelected() then
if m.categories.kids[msg.getindex()].title="Search" then
ShowMySearchScreen()
else
print "list item selected | index = "; msg.GetIndex()
kid = m.Categories.Kids[msg.GetIndex()]
if kid.type = "special_category" then
displaySpecialCategoryScreen()
else
displayCategoryPosterScreen(kid)
end if
end if
else if msg.isScreenClosed() then
return -1
end if
end If
end while
return 0
End Function
lock_4815162342
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
02-07-2014
12:52 PM
Re: SEARCH FUNCTIONALITY EXAMPLE?
Joel,
That was very helpful. It helped me get a much better understanding of how to manipulate the code.
Anyone,
I have been able to add a search screen to the channel. However, I am still a bit unclear about how I submit my search result to the server to find the content. I am using Amazon S3. Am I supposed to make a query to the parent URL that my files are on? Or is it a specific service that I acces somehow?
I am thankful for all the help so far. I feel like I am getting a much clearer understanding of Roku development.
That was very helpful. It helped me get a much better understanding of how to manipulate the code.
Anyone,
I have been able to add a search screen to the channel. However, I am still a bit unclear about how I submit my search result to the server to find the content. I am using Amazon S3. Am I supposed to make a query to the parent URL that my files are on? Or is it a specific service that I acces somehow?
I am thankful for all the help so far. I feel like I am getting a much clearer understanding of Roku development.