EnTerr
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
09-28-2016
03:51 PM
REP: roArray.slice(start, finish, step)
I propose an enhancement: add a .slice(start=0, finish=-1, step_=1) method for roArray , to return a new array that was sliced from the original (i.e. copied sub-range).
I know array slcing to be very useful and convenient, from my experience with Python - where there is even a syntactic sugar for that, the slice operator, e.g. [start:end], [start:end:step], [:], [::-1]. Especially if extending with negative notation to index backwards from the end of the array. For example:
Oh my - so powerful, perhaps it's too complicated writing the code to do all the acrobatics? Not at all - it is very easy to implement - see the sample implementation as B/S function i did couple of years ago viewtopic.php?f=34&t=75675#p461530 - it's only 10 lines! That one is fully-functional, except the speed is not that great since pushes&shoves non-natively. Slightly touched-up:
I know array slcing to be very useful and convenient, from my experience with Python - where there is even a syntactic sugar for that, the slice operator, e.g. [start:end], [start:end:step], [:], [::-1]. Especially if extending with negative notation to index backwards from the end of the array. For example:
lst = [0, 1, 2, 3, 4]
lst.slice(1, 3) '[1, 2, 3], from 1 to 3 - compare to "01234".mid(1, 3)'
lst.slice(3) '[2, 3, 4], from 2 onward - compare to "01234".mid(2)'
lst.slice(-3) '[2, 3, 4], last 3 - compare to "01234".right(3)'
lst.slice(-1, 0, -1) '[4, 3, 2, 1, 0], i.e. reversed, "from the end to the beginning, going backwards"'
lst.slice() '[0, 1, 2, 3, 4], array COPY - loosely akin to tmp=[]: tmp.append(lst): return tmp'
Oh my - so powerful, perhaps it's too complicated writing the code to do all the acrobatics? Not at all - it is very easy to implement - see the sample implementation as B/S function i did couple of years ago viewtopic.php?f=34&t=75675#p461530 - it's only 10 lines! That one is fully-functional, except the speed is not that great since pushes&shoves non-natively. Slightly touched-up:
function arraySlice(arr as Object, start=0 as Integer, finish=-1 as Integer, step_=1 as Integer) as Object:
if GetInterface(arr, "ifArray") = invalid then print "ValueError: arr not ifArray much" : STOP
if step_ = 0 then print "ValueError: slice step cannot be zero" : STOP
if start < 0 then start += arr.count() 'adjust, negative counts backwards from the end'
if finish < 0 then finish += arr.count()
res = []
for i = start to finish step step_:
res.push(arr[i])
next
return res
end function
5 REPLIES 5
EnTerr
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-19-2016
10:40 AM
Re: REP: roArray.slice(start, finish, step)
@Roku ?
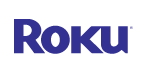

Roku Employee
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-24-2016
02:50 PM
Re: REP: roArray.slice(start, finish, step)
Feature request noted.
GarethJ
Reel Rookie
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-11-2020
07:16 AM
Re: REP: roArray.slice(start, finish, step)
So... 4 years later, how far have you gotten through adding those 10 lines?

Komag
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-18-2020
10:53 AM
Re: REP: roArray.slice(start, finish, step)
I guess since it's so easy to just code it as a Function there's not really a need for it.
GarethJ
Reel Rookie
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
12-15-2023
05:19 AM
Re: REP: roArray.slice(start, finish, step)
That applies to half of the API though. Yet that still exists.