robmurrer
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
01-03-2012
09:10 AM
Question about variable scope in Brightscript
Are all variables global?
In the videoplayer example I see the variable 'm' being used throughout different functions and I assume that it is a global.
In the videoplayer example I see the variable 'm' being used throughout different functions and I assume that it is a global.
4 REPLIES 4
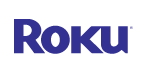
RokuMarkn
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
01-03-2012
09:49 AM
Re: Question about variable scope in Brightscript
See section 4.9 in the BrightScript Reference. All variables are local except for one special global variable, an AA, which is named m. Somewhat confusingly, m is also the name of a different variable, the "this" variable when a function is called through an AA member. In the latter case, the global m is not accessible, but you can get it via the GetGlobalAA function.
--Mark
--Mark
MazeWizzard
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
01-03-2012
11:34 AM
Re: Question about variable scope in Brightscript
I don't know if this helps... but maybe someone will benefit from it. Here's some code that I use to have global variables, local variables, etc. In the Main() function, I initialize the global variable pointer:
Then within any sub or function (including objects) that I want to have access to the global variables, I use g = GetGlobals(0) thus:
There's about 100 different ways to skin this cat. But if this helps.... cool. This stores all your "global" variables in the GlobalAA in a member called, surprisingly, "Globals". In the debugger you can always call "g=GetGlobals(0): ? g" if there is no local g assigned. Can call GetGlobals(0) inside any objects that need access too. I use g here, but you could use any variable (other than possibly m). Just make sure to have a local "g" reference if you need access to the globals. Don't ever pass it a -1 except the 1st time or it will wipe out your current globals. Also note that the GetGlobals() function must be at program scope (not made a member function of some other object.) This predates the GetGlobalAA() function (I think?), but still works fine. Had I returned "m" rather than "m.Globals" it would have been similar/equivalent to GetGlobalAA(). Meh.
Sub Main()
g = GetGlobals(-1) ' -1 only passed this once at start of main
g.BackgroundColor = &H000000FF
<rest of your code>
End Sub
Function GetGlobals(v as Integer) As Object
if v = -1 then
m.Globals = {}
end if
Return m.Globals
End Function
Then within any sub or function (including objects) that I want to have access to the global variables, I use g = GetGlobals(0) thus:
Sub Whatever()
g = GetGlobals(0)
g.BackgroundColor = g.BackgroundColor AND &H7F00 ' for example... accessing a global
End Sub
There's about 100 different ways to skin this cat. But if this helps.... cool. This stores all your "global" variables in the GlobalAA in a member called, surprisingly, "Globals". In the debugger you can always call "g=GetGlobals(0): ? g" if there is no local g assigned. Can call GetGlobals(0) inside any objects that need access too. I use g here, but you could use any variable (other than possibly m). Just make sure to have a local "g" reference if you need access to the globals. Don't ever pass it a -1 except the 1st time or it will wipe out your current globals. Also note that the GetGlobals() function must be at program scope (not made a member function of some other object.) This predates the GetGlobalAA() function (I think?), but still works fine. Had I returned "m" rather than "m.Globals" it would have been similar/equivalent to GetGlobalAA(). Meh.
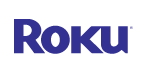
RokuMarkn
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
01-03-2012
03:53 PM
Re: Question about variable scope in Brightscript
There's nothing wrong with that code, however it would be simpler to just call GetGlobalAA in place of your GetGlobals function. That would store your variables directly in m, rather than m.Globals.
If you did want to use your technique, another simplification would be to do it like this so you don't have to pass a flag parameter:
--Mark
If you did want to use your technique, another simplification would be to do it like this so you don't have to pass a flag parameter:
Function GetGlobals() As Object
if m.Globals = invalid then m.Globals = {}
Return m.Globals
End Function
--Mark
MazeWizzard
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
01-03-2012
04:58 PM
Re: Question about variable scope in Brightscript
Thanks. Yeah, like I said... I think I developed it prior to hearing about GetGlobalAA() (or before it was added). However, I like your "If Invalid" simplification...avoids any mess ups. I'll incorporate that. THANK YOU.
Is the global AA used for anything else (like used by the components) or is it empty and for exclusive use by the currently running program? In other words, is there a chance of variable name conflicts external to my application? I guess I could go examine it, but don't know how to test for all possible uses of it so it's easier to just ask 😉
I'll probably just use the GetGlobalAA() routine for new code... or change it to just return "m".
Is the global AA used for anything else (like used by the components) or is it empty and for exclusive use by the currently running program? In other words, is there a chance of variable name conflicts external to my application? I guess I could go examine it, but don't know how to test for all possible uses of it so it's easier to just ask 😉
I'll probably just use the GetGlobalAA() routine for new code... or change it to just return "m".