dynamitemedia
Binge Watcher
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-27-2010
01:01 PM
Re: parsing XML question
endless i have it outside the next block now.... I actually did that after posting the code.
and your right its not getting all the values for some reason. if i just want one value it works but not for all of them even when its outside the for...
also it is only showing 1 result and not all 5 in the poster screen from the 5 in the xml file.
so what needs to change to get all those values i have and return all 5 of them as well?
thanks Mark, thats what i am trying to figure out, how would i go about doing that as well?
and your right its not getting all the values for some reason. if i just want one value it works but not for all of them even when its outside the for...
also it is only showing 1 result and not all 5 in the poster screen from the 5 in the xml file.
so what needs to change to get all those values i have and return all 5 of them as well?
thanks Mark, thats what i am trying to figure out, how would i go about doing that as well?
Twitter: iptvmyway facebook: iptvmyay
Channels: Warriors of War, Go Fight Live, Heading Outdoorz, IPTVmyway
Channels: Warriors of War, Go Fight Live, Heading Outdoorz, IPTVmyway
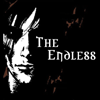
TheEndless
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-27-2010
01:09 PM
Re: parsing XML question
Without seeing the actual XML you're parsing, it's hard to say, but if you want to stick with your current method, my guess would be that you need two nested loops... One that loops through the song elements, and a second that loops through the song element's children elements...
Assuming this XML...
Your code should look something like this...
Of course, rather than loop through every child element, you could call them explicity:
but you probably need a bit more error handling around that to be safe.
Assuming this XML...
<songs>
<song>
<title>song title 1</title>
<url>song url 1</url>
</song
<song>
<title>song title 2</title>
<url>song url 2</url>
</song
<song>
<title>song title 3</title>
<url>song url 3</url>
</song
</songs>
Your code should look something like this...
For Each song in xmlSongs.song
'initialize variables with empty strings, just in case they're missing in the XML
title = ""
url = ""
For Each e in song.GetChildElements()
If e.GetName() = "title" Then
title = e.GetText()
End If
If e.GetName() = "url" Then
url = e.GetText()
End If
Next
song = CreateSong(title, url)
items.push(song)
Next
Of course, rather than loop through every child element, you could call them explicity:
title = song.title.GetText()
url = song.url.GetText()
but you probably need a bit more error handling around that to be safe.
My Channels: http://roku.permanence.com - Twitter: @TheEndlessDev
Instant Watch Browser (NetflixIWB), Aquarium Screensaver (AQUARIUM), Clever Clocks Screensaver (CLEVERCLOCKS), iTunes Podcasts (ITPC), My Channels (MYCHANNELS)
Instant Watch Browser (NetflixIWB), Aquarium Screensaver (AQUARIUM), Clever Clocks Screensaver (CLEVERCLOCKS), iTunes Podcasts (ITPC), My Channels (MYCHANNELS)
dynamitemedia
Binge Watcher
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-27-2010
01:11 PM
Re: parsing XML question
Ok let me go give that a whirl, i am having power outages over here cause of the windstorms so it has been hard testing
i will change the xml to that to be on the safe side... report back in a few 😉
i will change the xml to that to be on the safe side... report back in a few 😉
Twitter: iptvmyway facebook: iptvmyay
Channels: Warriors of War, Go Fight Live, Heading Outdoorz, IPTVmyway
Channels: Warriors of War, Go Fight Live, Heading Outdoorz, IPTVmyway
dynamitemedia
Binge Watcher
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-27-2010
02:20 PM
Re: parsing XML question
thanks i got it!!
had to make a few adjustments but got it...
Im gonna post the full code in case anyone else wants or needs to use it...
Thanks Endless and Jbrave
here is the finished code:
http://forums.roku.com/viewtopic.php?f=34&t=33396
maybe you can add some more error checking to it, seems to be working great!
had to make a few adjustments but got it...
Im gonna post the full code in case anyone else wants or needs to use it...
Thanks Endless and Jbrave
here is the finished code:
http://forums.roku.com/viewtopic.php?f=34&t=33396
maybe you can add some more error checking to it, seems to be working great!
Twitter: iptvmyway facebook: iptvmyay
Channels: Warriors of War, Go Fight Live, Heading Outdoorz, IPTVmyway
Channels: Warriors of War, Go Fight Live, Heading Outdoorz, IPTVmyway
dynamitemedia
Binge Watcher
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-27-2010
04:17 PM
Re: parsing XML question
So question for you endless...
I tried doing this same logic with the simplePoster example
i added this
but i am getting this error:
any idea why its saying that?
I tried doing this same logic with the simplePoster example
i added this
Function getShowsForCategoryItem(category As Object) as Object
m.UrlBase = "http://XXXX/roku"
m.UrlGetMp3Info = m.UrlBase + "/xml/mp3Playlist.php"
http = NewHttp(m.UrlGetMp3Info)
rsp = http.Http.GetToString()
xml = CreateObject("roXMLElement")
if not xml.Parse(rsp) then
print "Can't parse response"
ShowConnectionFailed()
return ""
end if
if xml.GetName() <> "songs"
Dbg("Bad register response: ", xml.GetName())
ShowConnectionFailed()
return ""
end if
if islist(xml.GetBody()) = false then
Dbg("No registration information available")
ShowConnectionFailed()
return ""
end if
For Each song in xml.song
'initialize variables with empty strings, just in case they're missing in the XML
title = ""
url = ""
image = ""
fmt = ""
desc = ""
author = ""
title = song.name.GetText()
url = song.url.GetText()
author = song.author.GetText()
image = song.image.GetText()
fmt = song.fmt.GetText()
desc = song.desc.GetText()
showList = [
{
ShortDescriptionLine1:title,
ShortDescriptionLine2:"Short Description for Show #2",
HDPosterUrl:image,
SDPosterUrl:image
}
]
Next
return showList
End Function
but i am getting this error:
/tmp/plugin/MJAAAALn5ldE/pkg:/source/SimplePoster.brs(169): runtime error e0: Function Call Operator ( ) attempted on non-function.
169: http = NewHttp(m.UrlGetMp3Info)
any idea why its saying that?
Twitter: iptvmyway facebook: iptvmyay
Channels: Warriors of War, Go Fight Live, Heading Outdoorz, IPTVmyway
Channels: Warriors of War, Go Fight Live, Heading Outdoorz, IPTVmyway
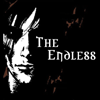
TheEndless
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-27-2010
05:02 PM
Re: parsing XML question
NewHttp is in urlUtils.brs which isn't part of the simplePoster example.
My Channels: http://roku.permanence.com - Twitter: @TheEndlessDev
Instant Watch Browser (NetflixIWB), Aquarium Screensaver (AQUARIUM), Clever Clocks Screensaver (CLEVERCLOCKS), iTunes Podcasts (ITPC), My Channels (MYCHANNELS)
Instant Watch Browser (NetflixIWB), Aquarium Screensaver (AQUARIUM), Clever Clocks Screensaver (CLEVERCLOCKS), iTunes Podcasts (ITPC), My Channels (MYCHANNELS)
dynamitemedia
Binge Watcher
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-27-2010
05:06 PM
Re: parsing XML question
man Endless ur so right i totally missed that!! geez let me add that and a few other things in there...
i'll test that in a few ...
i'll test that in a few ...
Twitter: iptvmyway facebook: iptvmyay
Channels: Warriors of War, Go Fight Live, Heading Outdoorz, IPTVmyway
Channels: Warriors of War, Go Fight Live, Heading Outdoorz, IPTVmyway
dynamitemedia
Binge Watcher
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-27-2010
09:51 PM
Re: parsing XML question
no matter what i do with this i can only get it to show one result...
EDIT: i even tried adding a extra for Loop and nothing still only getting one result, I checked my XML and its showing 3 results
EDIT: i even tried adding a extra for Loop and nothing still only getting one result, I checked my XML and its showing 3 results
Twitter: iptvmyway facebook: iptvmyay
Channels: Warriors of War, Go Fight Live, Heading Outdoorz, IPTVmyway
Channels: Warriors of War, Go Fight Live, Heading Outdoorz, IPTVmyway
- « Previous
- Next »