joetesta
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-17-2013
02:23 PM
array pass by value?
Hello -
I have an array (of shows) and I'm trying to create a parallel array that has one field different (no show description in 2nd array). So far whatever I try has the effect of altering both arrays. I tried setting newArray = oldArray, then when I modify the elements of newArray it also affects oldArray. I also tried looping over oldArray and Push'ing the elements to newArray, then loop over newArray and modify the field. Unfortunately oldArray's fields were also modified. It seems like there must be a way to do this without modifying the oldArray?
tyvmia
I have an array (of shows) and I'm trying to create a parallel array that has one field different (no show description in 2nd array). So far whatever I try has the effect of altering both arrays. I tried setting newArray = oldArray, then when I modify the elements of newArray it also affects oldArray. I also tried looping over oldArray and Push'ing the elements to newArray, then loop over newArray and modify the field. Unfortunately oldArray's fields were also modified. It seems like there must be a way to do this without modifying the oldArray?
tyvmia
aspiring
11 REPLIES 11
destruk
Streaming Star
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-17-2013
03:53 PM
Re: array pass by value?
That's weird Joe - one of those methods should work.
What seems to have worked for myself in the past is to create a new array entirely, grab values from the old array and push them individually to the new one, and then modify the new one, and use the new one for the new screen - that way the old array is never displayed except on the prior screen it came from. Unless the arrays are global, this should work all the time - but it's more work.
What seems to have worked for myself in the past is to create a new array entirely, grab values from the old array and push them individually to the new one, and then modify the new one, and use the new one for the new screen - that way the old array is never displayed except on the prior screen it came from. Unless the arrays are global, this should work all the time - but it's more work.
joetesta
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-17-2013
04:18 PM
Re: array pass by value?
thanks destruk! I think it happens because it's an array of objects; & I think i'd have to push the inner values of each object, rather than just pushing the objects themselves. But that's just my theory, i didn't go so far as to actually test that yet.
The goal is we want to hide the descriptions on the grid view but have them available on the detail view. The only way I could think to do this is to have parallel show lists, one with blank descriptions. I wonder if there's any other way to customize the grid view display...
The goal is we want to hide the descriptions on the grid view but have them available on the detail view. The only way I could think to do this is to have parallel show lists, one with blank descriptions. I wonder if there's any other way to customize the grid view display...
aspiring
destruk
Streaming Star
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-17-2013
04:21 PM
Re: array pass by value?
Yeah that's what I did before - create a new object entirely, push the values individually for that new object, and then push the object over. 😞
It might be a better idea to simply create a new array, and then re-download and re-parse the xml, and then make the change to the new array.
It might be a better idea to simply create a new array, and then re-download and re-parse the xml, and then make the change to the new array.
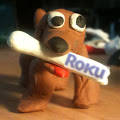
RokuJoel
Binge Watcher
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-17-2013
04:36 PM
Re: array pass by value?
An array is an object and even if you assign arrayB=arrayA you are still pointing at the same object. The only way to copy the array is to copy it one data item at a time by iterating over all elements in the array, as well as sub elements of those elements (for example if your array contains arrays).
- Joel
- Joel
joetesta
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-17-2013
05:01 PM
Re: array pass by value?
OK i went that route, create the new object and push the values in, then push the object onto the new array. Now it works, original array is unmodified. Rather than double the server requests for it. thanks!
aspiring
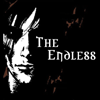
TheEndless
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-17-2013
07:04 PM
Re: array pass by value?
A quick array copy can be achieved by appending to a new array:
This will still have the issue of copying references to the inner objects to the new array, but it's a quicker way to break the top-level reference if using base types.
For deeper references, I use the following function which will copy all elements of an array or associative array down to the specified depth. A depth of 0, the default, does basically the same as the Append method above:
array1 = [
"value1"
"value2"
"value3"
]
array2 = []
array2.Append(array1)
This will still have the issue of copying references to the inner objects to the new array, but it's a quicker way to break the top-level reference if using base types.
For deeper references, I use the following function which will copy all elements of an array or associative array down to the specified depth. A depth of 0, the default, does basically the same as the Append method above:
Function ShallowCopy(array As Dynamic, depth = 0 As Integer) As Dynamic
If Type(array) = "roArray" Then
copy = []
For Each item In array
childCopy = ShallowCopy(item, depth)
If childCopy <> invalid Then
copy.Push(childCopy)
End If
Next
Return copy
Else If Type(array) = "roAssociativeArray" Then
copy = {}
For Each key In array
If depth > 0 Then
copy[key] = ShallowCopy(array[key], depth - 1)
Else
copy[key] = array[key]
End If
Next
Return copy
Else
Return array
End If
Return invalid
End Function
My Channels: http://roku.permanence.com - Twitter: @TheEndlessDev
Instant Watch Browser (NetflixIWB), Aquarium Screensaver (AQUARIUM), Clever Clocks Screensaver (CLEVERCLOCKS), iTunes Podcasts (ITPC), My Channels (MYCHANNELS)
Instant Watch Browser (NetflixIWB), Aquarium Screensaver (AQUARIUM), Clever Clocks Screensaver (CLEVERCLOCKS), iTunes Podcasts (ITPC), My Channels (MYCHANNELS)
destruk
Streaming Star
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-17-2013
11:47 PM
Re: array pass by value?
"Return invalid" will never be hit - so you can save a line there.
Thanks for posting that code.
Thanks for posting that code.
joetesta
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-18-2013
09:26 AM
Re: array pass by value?
"TheEndless" wrote:
A quick array copy can be achieved by appending to a new array
that append trick is very cool, exactly what i was looking for. Thanks!
aspiring

Komag
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
10-25-2017
11:12 AM
Re: array pass by value?
I realize this is years old, but I just can't believe this isn't an official Function by now! I use TheEndless' ShallowCopy() Function now in several key places to copy Associative Arrays of various depths where I don't want references but actual new copies.