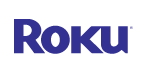
RokuKevin
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
02-03-2010
10:52 AM
Make your Springboard Screens Navigate to Next Item in Feed.
The SDK provides the APIs to enable the left & right remote keys to navigate to the next item in your feed. This is a convenient way for users to navigate the list of shows while viewing details about them.
The code below gives a simple example of how to enable this navigation.
The code below gives a simple example of how to enable this navigation.
'*************************************************************
'** LoadSpringboardContent()
'*************************************************************
Function LoadSpringboardContent(screen as object, items as object, index as integer)
item = items[index]
if item <> invalid and type(item) = "roAssociativeArray" and screen <> invalid and type(screen)= "roSpringboardScreen" then
screen.AllowUpdates(false)
screen.SetDescriptionStyle("video") 'audio, movie, video, generic
' generic+episode=4x3, generic+audio=audio
screen.ClearButtons()
screen.AddButton(1,"Play")
screen.AddRatingButton(2,80,20)
screen.AddButton(3,"Go Back")
screen.SetStaticRatingEnabled(false)
screen.AllowUpdates(true)
screen.SetContent(item)
screen.Show()
endif
End Function
'*************************************************************
'** showSpringboardScreenWithNav()
'*************************************************************
Function showSpringboardScreenWithNav(items as object, index as integer) As Integer
port = CreateObject("roMessagePort")
screen = CreateObject("roSpringboardScreen")
print "showSpringboardScreenWithNav"
screen.SetMessagePort(port)
LoadSpringboardContent(screen, items, index)
downKey=3
remoteLeft = 4
remoteRight = 5
selectKey=6
while true
msg = wait(0, screen.GetMessagePort())
if type(msg) = "roSpringboardScreenEvent"
if msg.isScreenClosed()
print "Screen closed"
exit while
else if msg.isButtonPressed()
print "Button pressed: "; msg.GetIndex(); " " msg.GetData()
else if msg.isRemoteKeyPressed()
print "Remote Key Pressed: "; msg.GetType(); " msg: "; msg.GetMessage();" index: "; msg.GetIndex(); " data: "; msg.GetData() " index: "; index " items.Count(): "; items.Count()
if msg.GetIndex() = remoteLeft then
if index > 0 then
index = index - 1
else if items.Count() > 0 then
index = items.Count() - 1
endif
LoadSpringboardContent(screen, items, index)
endif
if msg.GetIndex() = remoteRight then
if index < (items.Count() - 1) then
index = index + 1
else if (items.Count() - 1) = index then
index = 0
endif
LoadSpringboardContent(screen, items, index)
endif
else
print "Unknown event: "; msg.GetType(); " msg: "; msg.GetMessage()
endif
else
print "wrong type.... type=";msg.GetType(); " msg: "; msg.GetMessage()
endif
end while
return index
End Function