btpoole
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
11-09-2014
11:49 AM
Re: Help Parse XML
Thanks for looking over it. I am trying to create an array for the date, time, show, network and place them on a program guide that is organized by day. Sorry but I don't understand your reference of dictionary-inside-array thing. Not sure what that means. The idea is create canvas, push day, time, show, network to particular places on the canvas making up a program grid.
Thanks
Thanks
btpoole
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
11-11-2014
06:41 PM
Re: Help Parse XML
Thanks a million. Going back thru everthing once again I discovered something. If I leave out the xmlfilelist in the following, everything works as intended.
daylist=xmlfilelist.DAY@attr
If I use just
daylist=DAY@attr
all works.
Thanks again for looking over everything.
daylist=xmlfilelist.DAY@attr
If I use just
daylist=DAY@attr
all works.
Thanks again for looking over everything.
EnTerr
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
11-11-2014
08:06 PM
Re: Help Parse XML
I wrote a de-XML-izer for a case like yours, it "magically" converts roXml to a JSON-like structure. Don't know if it makes difference to you - but i'll share it here for posterity, perhaps it will help somebody:
Testing it on your feed:
Dumping as json It's not too readable but if i pretty-print it, here is what's inside xx:
And here is example of using the result:
PS. (11/13) fixed de_XML to not depend on roAA.count() existence (replaced res.count() < 1 with res.isEmpty() - which works for both roArray and roAA). Also updated example to avoid formatJSON(roArray). Both features are not in current fw5.6
function de_XML(xml):Feed it a roXmlElement after a .parse() and it returns array (ordered list) 1..N of elements, with leaf nodes folded together with attributes in [0]-th element.
attrs = xml.getAttributes()
res = [attrs]
chlds = xml.getChildElements(): if chlds = invalid then chlds = [ ]
for each child in chlds:
dex = de_XML(child)
if type(dex) = "String":
attrs[child.getName()] = dex
else:
res.push(dex)
end if
end for
if res.count() < 2 then res = attrs
if res.isEmpty() then res = xml.getText()
return res
end function
Testing it on your feed:
BrightScript Debugger> xfer = createObject("roUrlTransfer")
BrightScript Debugger> xfer.setURL("http://services.tvrage.com/feeds/fullschedule.php?country=US")
BrightScript Debugger> s = xfer.getToString(): ? len(s)
947987
BrightScript Debugger> x = createObject("roXmlElement")
BrightScript Debugger> x.parse(s)
BrightScript Debugger> xx = de_XML(x)
BrightScript Debugger> ss = formatJSON({_: xx}) : ? len(ss)
712524
BrightScript Debugger> ? left(ss, 1000)
{"_":[{},[{"attr":"2014-11-12"},[{"attr":"06:00 am"},{"ep":"17x285","link":"http://www.tvrage.com/Fox_and_Friends/episodes/1065649505","name":"Fox & Friends","network":"FOX NEWS channel","sid":"6978","title":"Season 17, Episode 285"},{"ep":"07x226","link":"http://www.tvrage.com/Mike_And_Mike_in_the_Morning/episodes/1065650083","name":"Mike & Mike","network":"ESPN 2","sid":"10566","title":"Season 7, Episode 226"},{"ep":"20x70","link":"http://www.tvrage.com/Squawk_Box/episodes/1065650146","name":"Squawk Box","network":"CNBC","sid":"18743","title":"Season 20, Episode 70"},{"ep":"10x28","link":"http://www.tvrage.com/Morning_Express_with_Robin_Meade/episodes/1065650204","name":"Morning Express with Robin Meade","network":"Headline News","sid":"19917","title":"Season 10, Episode 28"},{"ep":"08x08","link":"http://www.tvrage.com/ben-10-omniverse/episodes/1065703086","name":"Ben 10: Omniverse","network":"Cartoon Network","sid":"31489","title":"Most Dangerous Game Show"},{"ep":"02x156","link":"h
Dumping as json It's not too readable but if i pretty-print it, here is what's inside xx:
[from what originally was
{ },
[
{ attr: "2014-11-10" },
[
{ attr: "06:00 am" },
{
ep: "17x283",
link: "http:\/\/www.tvrage.com\/Fox_and_Friends\/episodes\/1065649503",
name: "Fox & Friends",
network: "FOX NEWS channel",
sid: "6978",
title: "Season 17, Episode 283"
},
{
ep: "01x46",
link: "http:\/\/www.tvrage.com\/bloomberg-surveillance\/episodes\/1065655054",
name: "Bloomberg Surveillance",
network: "Bloomberg TELEVISION",
sid: "44969",
title: "Season 1, Episode 46"
},
'...
],
[
{ attr: "07:00 am" },
'...
],
' ...
],
[
{ attr: "2014-11-11" },
[
{ attr: "06:00 am" },
'...
]
' ...
]
]
<?xml version="1.0" encoding="UTF-8" ?>
<schedule>
<DAY attr="2014-11-10">
<time attr="06:00 am">
<show name="Fox & Friends">
<sid>6978</sid>
<network>FOX NEWS channel</network>
<title>Season 17, Episode 283</title>
<ep>17x283</ep>
<link>http://www.tvrage.com/Fox_and_Friends/episodes/1065649503</link>
</show>
<show name="Bloomberg Surveillance">
<sid>44969</sid>
<network>Bloomberg TELEVISION</network>
<title>Season 1, Episode 46</title>
<ep>01x46</ep>
<link>http://www.tvrage.com/bloomberg-surveillance/episodes/1065655054</link>
</show>
' ...
</time>
<time attr="07:00 am">
' ...
</time>
'...
</DAY>
<DAY attr="2014-11-11">
<time attr="06:00 am">
'...
</DAY>
'...
<schedule>
And here is example of using the result:
function dump(schedule):
for i = 1 to 2 'schedule.count()-1:
day_sched = schedule[i]
day = day_sched[0].attr
for j = 1 to 2 'day_sched.count()-1:
hour_sched = day_sched[j]
time = hour_sched[0].attr
for k = 1 to 2 'hour_sched.count()-1:
? day, time, hour_sched[k].name
end for
end for
end for
end function
BrightScript Debugger> dump(xx)(Here for brevity i printed just the first 2 shows from the first 2 hours from the first 2 days)
2014-11-10 06:00 am Fox & Friends
2014-11-10 06:00 am Mike & Mike
2014-11-10 07:00 am Good Morning America
2014-11-10 07:00 am Today (US)
2014-11-11 06:00 am Fox & Friends
2014-11-11 06:00 am Mike & Mike
2014-11-11 07:00 am Good Morning America
2014-11-11 07:00 am Today (US)
PS. (11/13) fixed de_XML to not depend on roAA.count() existence (replaced res.count() < 1 with res.isEmpty() - which works for both roArray and roAA). Also updated example to avoid formatJSON(roArray). Both features are not in current fw5.6
btpoole
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
11-12-2014
04:49 PM
Re: Help Parse XML
EnTerr
You have exceeded all wishes. Thank you a million times over for your help on this. Now, I am having one issue. I keep getting an error on the line:
if res.count() < 1 then res= xml.getText()
Bugger keeps telling me member of function not found in Brightscript Component or interface. I am think it is the xml.getText() but not sure.
Thanks again
You have exceeded all wishes. Thank you a million times over for your help on this. Now, I am having one issue. I keep getting an error on the line:
if res.count() < 1 then res= xml.getText()
Bugger keeps telling me member of function not found in Brightscript Component or interface. I am think it is the xml.getText() but not sure.
Thanks again
EnTerr
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
11-12-2014
06:42 PM
Re: Help Parse XML
"btpoole" wrote:
I keep getting an error on the line:if res.count() < 1 then res= xml.getText()Bugger keeps telling me member of function not found in Brightscript Component or interface. I am think it is the xml.getText() but not sure.
When you get that error, it breaks into console, right?
Just see what's in "res" and "xml" and that will give you idea what's going on. Either by typing "var" or "? type(xml), xml" (sans quotes) and so on...
I cannot guess a reason for getting such error - to get to that line it has gone through xml.getAttributes() and xml.getChildElements() earlier in the function w/o error, so i suppose xml was roXmlElement type and must have getString(). You called it with roXmlElement and not roXmlList - right?
PS. Oh. OH! I KNOW! You must have called it with a roXmlList, then "attrs = xml.getAttributes()" would have seeded attrs with invalid and that got assigned to res in previous line, hence the error here since there is no invalid.count(). Don't call it with roXmlList, it's not written for that (i can probably expand it but let's take it easy).
btpoole
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
11-13-2014
04:38 AM
Re: Help Parse XML
xml = CreateObject("roXMLElement")
I kinda that it was all good since it got thru the attributes but it kicks on res.count < 1 line. It breaks to the console as you thought. When I ?type(xml) I get nothing back.
I kinda that it was all good since it got thru the attributes but it kicks on res.count < 1 line. It breaks to the console as you thought. When I ?type(xml) I get nothing back.
malort
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
11-13-2014
09:45 AM
Re: Help Parse XML
It's due to this line
Not sure what was expected with the code, but that will set res to an roAssociativeArray, causing the next line to break.
if res.count() < 2 then res = attrs
Not sure what was expected with the code, but that will set res to an roAssociativeArray, causing the next line to break.
EnTerr
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
11-13-2014
09:57 AM
Re: Help Parse XML
"malort" wrote:
It's due to this lineif res.count() < 2 then res = attrsNot sure what was expected with the code, but that will set res to an roAssociativeArray, causing the next line to break.
Uh, no.
It may set res to AA but AA's have .count(), so not causing next line to break.
And roXMLs have .getText() (it's "xml" there, not "res").
malort
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
11-13-2014
10:11 AM
Re: Help Parse XML
AA does not have a .count()
heh, better example instead of setting count..
BrightScript Debugger> aa = createObject("roAssociativeArray")
BrightScript Debugger> aa["count"] = "no"
BrightScript Debugger> ?aa.count()
Function Call Operator ( ) attempted on non-function. (runtime error &he0) in $LIVECOMPILE(211)
heh, better example instead of setting count..
BrightScript Debugger> aa = createObject("roAssociativeArray")
BrightScript Debugger> aa["what"] = "no"
BrightScript Debugger> ?aa.count()
Member function not found in BrightScript Component or interface. (runtime error &hf4) in $LIVECOMPILE(221)
BrightScript Debugger> aa = {}
BrightScript Debugger> ?aa.count()
Member function not found in BrightScript Component or interface. (runtime error &hf4) in $LIVECOMPILE(224)
BrightScript Debugger> a = []
BrightScript Debugger> ?a.count()
0
"EnTerr" wrote:"malort" wrote:
It's due to this lineif res.count() < 2 then res = attrsNot sure what was expected with the code, but that will set res to an roAssociativeArray, causing the next line to break.
Uh, no.
It may set res to AA but AA's have .count(), so not causing next line to break.
And roXMLs have .getText() (it's "xml" there, not "res").
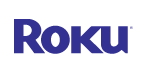
RokuMarkn
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
11-13-2014
10:26 AM
Re: Help Parse XML
EnTerr, what firmware version are you running? AAs do not have a count() method in 5.6, but you may see it if you're running later beta firmware.
--Mark
--Mark