ShifterFilms
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
06-30-2012
10:02 PM
Device linking with a WordPress user database
We need to have our users who sign up via a WordPress site using Digital Access Pass as our member management system be able to link their Roku devices to their account with us. Can someone point me in the right direction? Anybody out there have experience with WordPress and/or Digital Access Pass and device linking?
11 REPLIES 11
Trevor
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
07-01-2012
12:46 PM
Re: Device linking with a WordPress user database
On the Roku end you'll need to allow you users to enter their username and password and then on the server end a script that the Roku accesses and checks to database if that user is signed up. Alternately you can do the whole access code thing where the user is given a code by the Roku and then must enter it into their account on the WordPress site to link them. You'll still need the Roku to hit a script on your server that will check the database for their access code to see if the account is active.
*** Trevor Anderson - bloggingwordpress.com - moviemavericks.com ***
ShifterFilms
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
07-02-2012
12:34 PM
Re: Device linking with a WordPress user database
Hi - Thank you for the reply, Trevor.
I know the ways it can be done, I'm looking for the how to get it done. From the Roku side, are there examples in the SDK of both types of authentication?
From the WordPress side, I'm interested in finding someone who can help us with what needs to be done. Maybe it will just be apparent if I can see how it works with an example from the SDK...
I know the ways it can be done, I'm looking for the how to get it done. From the Roku side, are there examples in the SDK of both types of authentication?
From the WordPress side, I'm interested in finding someone who can help us with what needs to be done. Maybe it will just be apparent if I can see how it works with an example from the SDK...
ShifterFilms
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
07-11-2012
06:19 PM
Re: Device linking with a WordPress user database
I've made a lot of progress with this on my own. I'll try to post my results once it is finished. I need a couple of pointers, though...
Does anyone have an example of the PHP script used to authenticate the token with a server after device linking? And, more importantly because I can probably figure that part out, where in the Register example in the SDK do you define the script used to authenticate the token in the future?
Does anyone have an example of the PHP script used to authenticate the token with a server after device linking? And, more importantly because I can probably figure that part out, where in the Register example in the SDK do you define the script used to authenticate the token in the future?
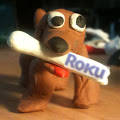
RokuJoel
Binge Watcher
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
07-11-2012
06:25 PM
Re: Device linking with a WordPress user database
I posted some rough examples a while back, in this thread:
viewtopic.php?f=34&t=34279&hilit=php%27#p321080
viewtopic.php?f=34&t=34279&hilit=php%27#p321080
ShifterFilms
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
07-11-2012
09:47 PM
Re: Device linking with a WordPress user database
Hey Joel -
I used some of your code to get me this far! Thanks so much for posting that, it was very helpful early on.
Where I'm stuck now is the validation of the token. I'm trying to use this code in the BrightScript:
It is slightly modified from another post I found, but I can't seem to get the script right which would validate this on the server. I know the XML response needs to be
But what does the PHP look like to process the info sent from the BrightScript to the server script that produces the pass or fail response? I feel like I'm soooooo close, I just can't figure this last bit out.
I used some of your code to get me this far! Thanks so much for posting that, it was very helpful early on.
Where I'm stuck now is the validation of the token. I'm trying to use this code in the BrightScript:
Function isLinked() As Dynamic
if Len(m.RegToken) > 0 then
' send RegToken to server for validation
http = NewHttp(m.UrlValidate)
http.AddParam("RegToken", m.RegToken)
rsp = http.Http.GetToString()
xml = CreateObject("roXMLElement")
print "GOT: " + rsp
print "Reason: " + http.Http.GetFailureReason()
if not xml.Parse(rsp) then
print "Can't parse getRegistrationCode response"
ShowConnectionFailed()
return ""
endif
if xml.GetName() <> "result"
Dbg("Bad register response: ", xml.GetName())
ShowConnectionFailed()
return ""
endif
if islist(xml.GetBody()) = false then
Dbg("No registration information available")
ShowConnectionFailed()
return ""
endif
'set default value for validate
validate = "fail"
'handle validation of response fields
for each e in xml.GetBody()
if e.GetName() = "validate" then
validate = e.GetBody() 'pass or fail
endif
next
if validate = "" then
Dbg("Parse yields empty validation result")
ShowConnectionFailed()
endif
if validate = "pass" then return true
endif
return false
End Function
It is slightly modified from another post I found, but I can't seem to get the script right which would validate this on the server. I know the XML response needs to be
<result>
<validate>pass</validate>
</result>
But what does the PHP look like to process the info sent from the BrightScript to the server script that produces the pass or fail response? I feel like I'm soooooo close, I just can't figure this last bit out.
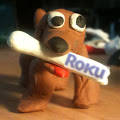
RokuJoel
Binge Watcher
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
07-12-2012
10:24 AM
Re: Device linking with a WordPress user database
I'm just checking this script, if it fails to give me a code then the user has not entered it and it has not been stored in the database. If it doesn't give me a code, then it has failed, otherwise it has succeeded. A success returns the code, which is then stored in the roku's registry and either a) the application is considered "authorized" and that is it, or b) you can include the code as a url parameter on every API call and validate that it is a legitimate code.
<?
// Define settings
$dbserver = "mysql"; // Change as required
$dbname = "mydatabase"; // Whatever DB you have access to
$dbuser = "myusername"; // User to connect to DB as
$dbpass = "mypassword";// Password for DB user
$code="";
// Connect to DB
$dbconn = mysql_connect("mysql","myusername","mypassword");
if (!$dbconn) die("Error connecting to database!");
if (!mysql_select_db($dbname)) die("Error selecting database $dbname");
// Get args
$code = !empty($_REQUEST['code']) ? mysql_real_escape_string($_REQUEST['code']) : "";
// Verify args
if (empty($code)) die("Missing or invalid Code");
$sql = "SELECT * FROM `users` WHERE code =\"" . $code."\"";
//echo "sql follows";
//echo "<br>";
//echo $sql;
// Get a specific result from the "example" table
$result = mysql_query($sql) or die(mysql_error());
// get the first (and hopefully only) entry from the result
$row = mysql_fetch_array($result);
// Print out the contents of each row into a table
echo $row['code'];
?>
ShifterFilms
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
07-12-2012
04:39 PM
Re: Device linking with a WordPress user database
Thanks to Joel for pointing me in the right direction! I'd like to share my code. The BrightScript I posted above is unchanged. Here is the PHP script which will authenticate a token you've written to your database. Please note, this script assumes that the mysql row you've stored the user's token is associated with that user. Basically, if the token is in the database, this script will ensure a previously validated user passes authentication and gets to the content they have paid for.
<?php
$con = mysql_connect("localhost","user","password");
if (!$con)
{
die('Could not connect: ' . mysql_error());
}
mysql_select_db("database_name", $con);
$regToken = $_GET['RegToken'];
$sql = mysql_query("select 1 from reg_tokens where regToken = '$regToken'");
$row = mysql_fetch_array($sql);
if ( ! mysql_num_rows($sql)) {
$status = 'fail';
} else {
$status = 'pass';
}
?>
<result>
<validate><?php echo $status; ?></validate>
</result>
atheling
Binge Watcher
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
07-12-2012
07:55 PM
Re: Device linking with a WordPress user database
"ShifterFilms" wrote:
Thanks to Joel for pointing me in the right direction! I'd like to share my code. The BrightScript I posted above is unchanged. Here is the PHP script which will authenticate a token you've written to your database. Please note, this script assumes that the mysql row you've stored the user's token is associated with that user. Basically, if the token is in the database, this script will ensure a previously validated user passes authentication and gets to the content they have paid for.
<?php
$con = mysql_connect("localhost","user","password");
if (!$con)
{
die('Could not connect: ' . mysql_error());
}
mysql_select_db("database_name", $con);
$regToken = $_GET['RegToken'];
$sql = mysql_query("select 1 from reg_tokens where regToken = '$regToken'");
$row = mysql_fetch_array($sql);
if ( ! mysql_num_rows($sql)) {
$status = 'fail';
} else {
$status = 'pass';
}
?>
<result>
<validate><?php echo $status; ?></validate>
</result>
You can register an action with Wordpress for "init" which will get called after Wordpress sets up its database object but before anything is output for the request. In that action you can check for the token validation/authentication request and if that's what it is then output the validation and exit. That means you don't have to futz with opening the database yourself and you can make your Wordpress plugin (I assume that is how you are implementing this) compatible on its database access. And you can use your URL for your Wordpress based site without having to worry about conflicts with page names, etc.
Also, even in this trivial case, just passing a get parameter to your select query is opening you up to SQL injection attacks....
ShifterFilms
Visitor
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
07-14-2012
01:04 PM
Re: Device linking with a WordPress user database
"atheling" wrote:
You can register an action with Wordpress for "init" which will get called after Wordpress sets up its database object but before anything is output for the request. In that action you can check for the token validation/authentication request and if that's what it is then output the validation and exit. That means you don't have to futz with opening the database yourself and you can make your Wordpress plugin (I assume that is how you are implementing this) compatible on its database access. And you can use your URL for your Wordpress based site without having to worry about conflicts with page names, etc.
Also, even in this trivial case, just passing a get parameter to your select query is opening you up to SQL injection attacks....
I'm actually not using a plug-in. I'd like to look more into your solution, but I'm not familiar with init.
Can you explain the vulnerability to SQL injection attacks? I'm not making the URLs publicly available, so I'm curious as to where the vulnerability would lie.