EnTerr
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
08-12-2014
03:27 PM
Checking if FOR was EXIT-ed
I have a FOR loop, which i may EXIT or not - and further after that i need to know if it finished prematurely or by exhausting the range. In example, i came up with this:
By reading the manual, it seems to confirm my expectations: "When program flow reaches the END FOR statement, the counter is incremented by the amount specified in the STEP increment" - that is, it will increment once more after the last value before exiting, so i should be good by checking if out of bound.
The reason i have any doubt is - in other languages (maybe even BASIC) i remember spec saying something akin to "value of iterator i is undefined after exiting the for loop". So someone knowing the matter (RokuMarkN?), can you relieve my anxiety by saying if my code assumption is good?
for i = stack.count()-1 to 0 step -1:
if stack[i] = myVal then exit for
end for
if i < 0 then ... 'not found
By reading the manual, it seems to confirm my expectations: "When program flow reaches the END FOR statement, the counter is incremented by the amount specified in the STEP increment" - that is, it will increment once more after the last value before exiting, so i should be good by checking if out of bound.
The reason i have any doubt is - in other languages (maybe even BASIC) i remember spec saying something akin to "value of iterator i is undefined after exiting the for loop". So someone knowing the matter (RokuMarkN?), can you relieve my anxiety by saying if my code assumption is good?
5 REPLIES 5
belltown
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
08-12-2014
07:06 PM
Re: Checking if FOR was EXIT-ed
I'm pretty sure your assumptions are safe based on the following statements in the Brightscript Reference Manual, Section 4.9, Scope:
- Local variables exist with function Scope
- Block Statements (like FOR-END FOR or WHILE-END WHILE) do not create a separate scope
From personal experience also, this appears to be true. The languages you alluded to that specify that the value of an iterator is undefined after exiting a for-loop, create the loop counter within a separate scope. In the following C statement, for example, if "i" is declared within the 'for' statement then it will be out of scope after the loop block: for (i = stack.count()-1; i <= 0; i--) { .... }
- Local variables exist with function Scope
- Block Statements (like FOR-END FOR or WHILE-END WHILE) do not create a separate scope
From personal experience also, this appears to be true. The languages you alluded to that specify that the value of an iterator is undefined after exiting a for-loop, create the loop counter within a separate scope. In the following C statement, for example, if "i" is declared within the 'for' statement then it will be out of scope after the loop block: for (i = stack.count()-1; i <= 0; i--) { .... }
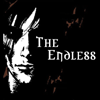
TheEndless
Channel Surfer
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
08-12-2014
08:08 PM
Re: Checking if FOR was EXIT-ed
Any reason you can't err on the side of caution and use a flag to indicate whether you exited early or not?
My Channels: http://roku.permanence.com - Twitter: @TheEndlessDev
Instant Watch Browser (NetflixIWB), Aquarium Screensaver (AQUARIUM), Clever Clocks Screensaver (CLEVERCLOCKS), iTunes Podcasts (ITPC), My Channels (MYCHANNELS)
Instant Watch Browser (NetflixIWB), Aquarium Screensaver (AQUARIUM), Clever Clocks Screensaver (CLEVERCLOCKS), iTunes Podcasts (ITPC), My Channels (MYCHANNELS)
EnTerr
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
08-12-2014
10:43 PM
Re: Checking if FOR was EXIT-ed
"TheEndless" wrote:
Any reason you can't err on the side of caution and use a flag to indicate whether you exited early or not?
You mean is there any use to it besides my misguided affection for beautiful code/code poetry?
Well I like to "make things as simple as possible - but not simpler". I can use an extra flag or a "sentinel" value (say in my example could plant myVal as stack[0] to ensure it will always EXIT-FOR). But that'd add verbosity to the code and the more baroque something is, more difficult to troubleshoot and maintain. Right now i have ~3/4 of xml parser* ready (TBD are <!--, <![CDATA[ and &entity; expansion) - and it fits on two screens (<100 lines) so far. Some complicated branching happens and I need to be able to easily read my code to fit all in my head. This justifies my motivation for brevity.
(*) seems to me at least half the regulars in this forum have written their own xml/html parser at some point, for one reason or another
destruk
Streaming Star
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
08-12-2014
10:56 PM
Re: Checking if FOR was EXIT-ed
Well your code idea works fine - it will exit with "-1" if nothing was found just like you wanted. I'd still use a flag variable regardless just for easier legibility when I forget where the value came from.
Otherwise it might make more sense from a debugging standpoint if you were to do it like this.
Otherwise it might make more sense from a debugging standpoint if you were to do it like this.
for i = stack.count() to 1 step -1:
if stack[i-1] = myVal then exit for
end for
if i = 0 then ... 'not found
belltown
Roku Guru
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
08-12-2014
11:25 PM
Re: Checking if FOR was EXIT-ed
I can see why you're doing what you're doing, although trying to read your code (and destruk's) makes my head hurt.
So I'd have to agree with the others that a flag might make for cleaner, easier-to-debug, easier-to-maintain code:
No loop counters (and therefore no scope worries if that's something that concerns you), no start/end condition errors, no worrying about whether your loops should be starting/ending/incrementing/decrementing by 1 or zero or -1, or if your test condition should be "< 0", "= 0", "<= 0", " < 1" etc. I suppose it would still be possible to make mistakes (like get the trues and falses) mixed up, but I think that kind of error would be easier to spot.
Although if you have to search the stack backwards, like in your example, then you'll probably have to end up using a loop counter anyway, although I'd do something like:
So I'd have to agree with the others that a flag might make for cleaner, easier-to-debug, easier-to-maintain code:
found = false
for each item in stack
if item = myval
found = true
exit for
endif
end for
if not found
' Not found ...
endif
No loop counters (and therefore no scope worries if that's something that concerns you), no start/end condition errors, no worrying about whether your loops should be starting/ending/incrementing/decrementing by 1 or zero or -1, or if your test condition should be "< 0", "= 0", "<= 0", " < 1" etc. I suppose it would still be possible to make mistakes (like get the trues and falses) mixed up, but I think that kind of error would be easier to spot.
Although if you have to search the stack backwards, like in your example, then you'll probably have to end up using a loop counter anyway, although I'd do something like:
found = false
i = stack.Count () - 1 ' Last item
while not found and i >= 0 ' Be sure to examine first item (0)
if stack [i] = myval
found = true
else
i = i - 1
endif
end while